Ring Modulation
This tutorial shows raw modulation.
It shows what happens when the output of one waveform is directly used
as the amplitude for another.
Hear the result of this tutorial's
music below.
View / Download
source - Instrument source
code
View / Download source - Testing source code
Instrument
Here is the code:
import jm.audio.io.*; import jm.audio.synth.*; import jm.music.data.Note; import jm.audio.AudioObject; public final class RingModulationInst extends jm.audio.Instrument{ / private int sampleRate; private int channels; public RingModulationInst(int sampleRate){ this.sampleRate = sampleRate; this.channels = 1; } public void createChain(){ Oscillator modulator = new Oscillator(this, Oscillator.SINE_WAVE, this.sampleRate, this.channels); modulator.setFrqRatio((float)2.1); Envelope env = new Envelope(modulator, new double[] {0.0, 0.0, 1.0, 1.0}); Oscillator carrier = new Oscillator(env, Oscillator.SINE_WAVE, Oscillator.AMPLITUDE); Envelope env2 = new Envelope(carrier, new double[] {0.0, 0.0, 0.05, 1.0, 1.0, 0.0}); SampleOut sout = new SampleOut(env2); } }
|
Ring Modulation
This instrument alters (modulates) the
amplitude of one waveform (the carrier) by the sample values of a second
(the modulator). Ring modulation is a simple multiplication of one
waveform amplitude values by another. In ring modulation the modulator
output is applied directly to the input of the carrier, without any DC
offset to remove negative values as done in ‘classic’ ring modulation.
Ring modulation creates a somewhat unpredictable sound, often
clangourous, because neither the frequency of the carrier or modulator
appear in the final output, instead sidebands (overtones) at the sum and
difference frequencies appear. In this example sine waves are used. You
can imagine that when more complex sounds are used the resulting timbre
is even more complex. |
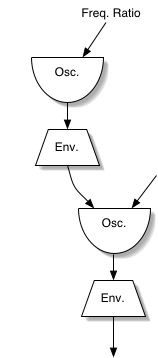 |
Composition
Example
The
following example creates a note using the RingModulationInst and
outputs an audio file of it.
import jm.JMC; import jm.music.data.*; import jm.audio.*; import jm.util.*; public final class RingModTest implements JMC{ public static void main(String[] args){ Score score = new Score("JMDemo - Audio test"); Part part = new Part("wave", 0); Phrase phr = new Phrase(0.0); int sampleRate = 44100; Instrument inst = new RingModulationInst(sampleRate); Note note = new Note(C4, 4.0); phr.addNote(note); part.addPhrase(phr); score.addPart(part); Write.au(score, "RingModTest.au", inst); } }
|
|